Have you ever wanted to save time and eliminate the process of recreating repetitive code for apps, plugins, or other needs?
Boilerplate code is the perfect way to build software and other applications. It provides the foundation and allows for the reuse of elements without the need to write the code yourself.
In this article, we are going to explain what is boilerplate code, and how it benefits programmers, who wish to invest time in refining code, and building more features.
What is boilerplate code?
Boilerplate codes are reusable code components, which can be used without modifications. The sole purpose of boilerplate codes is to reduce the time of rewriting repetitive code and use your time to create more customized solutions for your or your client’s business.
By utilizing boilerplate code listing sites, you will find premade boilerplates for all the popular programming languages, which could help you create unique solutions in more than one language.
If you know a programming language and wish to create projects instantly, then boilerplates are the perfect way to start building.
However, for newbies, boilerplates are not a recommended solution, since their understanding of how code works could interfere with building an application or software further.
If you are a beginner in programming, learning the fundamentals and understanding the logic of the code, could help you use boilerplate code further on.
As you may know, boilerplates could help experienced programmers focus their time on building unique features, while eliminating repetitiveness in already existing components. To further simplify your process, it is a good practice to integrate Portainer with Docker, which allows you to manage your boilerplate code efficiently.
Boilerplate code examples
To further help you understand what a boilerplate code is, we have outlined a few examples of popular programming languages, which represent a function.
Java boilerplate example
Here’s how a Java boilerplate code will look when working on a project.
// Import necessary packages
import java.util.Scanner;
public class Main {
// Main method - entry point of the program
public static void main(String[] args) {
// Create a scanner object to take input from the user
Scanner scanner = new Scanner(System.in);
// Print a message to the user
System.out.println("Welcome to the Java Boilerplate Program!");
// Example: Asking for the user's name and displaying a greeting
System.out.print("Please enter your name: ");
String name = scanner.nextLine();
// Display a greeting message
System.out.println("Hello, " + name + "! Welcome to the Java world.");
// Close the scanner object
scanner.close();
}
}
Javascript boilerplate example
Here’s how a JavaScript boilerplate code will look when working on a project.
// Basic JavaScript Boilerplate Code
// Function to handle user input and display a greeting message
function greetUser() {
// Prompt the user for their name
let name = prompt("Please enter your name:");
// Check if a name was entered and display the greeting
if (name) {
alert("Hello, " + name + "! Welcome to the JavaScript world.");
} else {
alert("Hello, stranger! Welcome to the JavaScript world.");
}
}
// Call the function to greet the user
greetUser();
PHP boilerplate example
Here’s how a PHP boilerplate code will look when working on a project.
<?php
// Basic PHP Boilerplate Code
// Function to handle user input and display a greeting message
function greetUser() {
// Check if the form is submitted
if (isset($_POST['submit'])) {
// Get the user's name from the form input
$name = $_POST['name'];
// Display a greeting message if a name is provided
if (!empty($name)) {
echo "Hello, " . htmlspecialchars($name) . "! Welcome to the PHP world.";
} else {
echo "Hello, stranger! Welcome to the PHP world.";
}
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PHP Boilerplate</title>
</head>
<body>
<!-- Form to take user input -->
<form method="POST" action="">
<label for="name">Please enter your name:</label><br>
<input type="text" id="name" name="name" required><br><br>
<input type="submit" name="submit" value="Submit">
</form>
<?php
// Call the function to greet the user after form submission
greetUser();
?>
</body>
</html>
Python boilerplate example
Here’s how a Python boilerplate code will look when working on a project.
# Basic Python Boilerplate Code
def greet_user():
# Get the user's name
name = input("Please enter your name: ")
# Check if a name was entered and display the greeting
if name:
print(f"Hello, {name}! Welcome to the Python world.")
else:
print("Hello, stranger! Welcome to the Python world.")
# Call the function to greet the user
greet_user()
HTML boilerplate example
Here’s how a HTML boilerplate code will look when working on a project.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Boilerplate</title>
<style>
body {
font-family: Arial, sans-serif;
padding: 20px;
}
label, input {
margin: 10px 0;
}
</style>
</head>
<body>
<h1>Welcome to the HTML Boilerplate</h1>
<p>Please enter your name:</p>
<!-- Form to take user input -->
<form id="greetForm">
<label for="name">Your Name:</label><br>
<input type="text" id="name" name="name" required><br><br>
<button type="submit">Submit</button>
</form>
<p id="greetingMessage"></p>
<script>
// Function to handle form submission and display greeting
document.getElementById("greetForm").addEventListener("submit", function(event) {
event.preventDefault(); // Prevent page refresh
let name = document.getElementById("name").value;
let greetingMessage = document.getElementById("greetingMessage");
if (name) {
greetingMessage.textContent = "Hello, " + name + "! Welcome to the HTML world.";
} else {
greetingMessage.textContent = "Hello, stranger! Welcome to the HTML world.";
}
});
</script>
</body>
</html>
These examples, help with publishing projects, such as websites built with boilerplate code.
What are the benefits of boilerplate code?
You can find many benefits of using boilerplate code, especially, if you are a senior programmer, who wants to cut off time programming the same components and build unique features.
Time Efficiency
Boilerplate code saves time by providing a ready-made structure, so you don’t have to start from scratch every time.
Instead of writing the same basic setup for each project, it allows you to focus on the unique aspects of the application.
Consistency
Consistency is another major advantage. When using boilerplate code, the structure and format are standardized across the team or organization.
This ensures that everyone is working within the same framework, leading to fewer errors and greater harmony in the structure.
Standard practices, like naming conventions or directory structures, make the entire project easier to manage and understand.
Collaboration
Collaboration benefits significantly from boilerplate code. Since the code structure is familiar to everyone, team members can more easily jump into tasks without having to learn the entire setup.
New developers, in particular, can get up to speed faster as they don’t have to start from zero and can focus on understanding the specific business logic of the project.
Error Prevention
By providing a foundation with built-in best practices, boilerplate code minimizes mistakes. For example, common security practices and efficient coding patterns are often included.
These features reduce the chance of bugs and vulnerabilities that might occur if developers had to implement them individually each time.
Rapid Prototyping
Finally, boilerplate code accelerates prototyping and development. It allows developers to quickly put together a working prototype or MVP, which is critical for testing ideas or meeting tight deadlines.
With less time spent on basic setup, developers can prioritize the actual product functionality and fine-tune their application faster.
Understanding the difference between Boilerplate code and custom code
Now that you know about boilerplate code, it is utterly important to understand when you need to use boilerplate code and custom code.
The primary difference between boilerplate and custom code lies in their purpose and flexibility.
For example, boilerplate is designed for quick, standardized setups across various projects, while custom code is tailored to meet the specific needs and goals of an individual project.
Boilerplate often lacks the flexibility to handle unique requirements, and developers are required to modify or extend it with custom code to achieve the desired functionality.
On the other hand, custom code offers the freedom to implement unique features and optimize performance, but requires more effort to write, test, and maintain.
Depending on your situation, boilerplate code can accelerate the starting stages of development, by providing a reusable framework.
However, custom code can provide specific solutions, such as full control over the behavior and functionality of an application.
Both can be essential in different contexts and can be used together to balance efficiency and customization.
When to use boilerplate code?
Boilerplate code is ideal when starting a new project, especially with established frameworks, as it provides the foundational structure and saves time.
It’s useful when working with common frameworks, building prototypes or MVPs, and collaborating in teams where consistency is key.
Boilerplate also helps when creating reusable components for future projects or working on large-scale applications by organizing complex structures.
When not to use boilerplate code?
In the same case, boilerplate code should be avoided when working on highly unique or specialized projects that require custom solutions.
When working with custom and feature-rich applications, boilerplate code can often limit the ability to customize further.
It should also be avoided in situations where performance is a critical concern, as boilerplate often contains unnecessary or generic elements that could affect efficiency.
The difference between Boilerplate code and Templates
Boilerplate code and templates both serve as starting points for development, but they have distinct differences in purpose and usage.
As you now know, boilerplate code refers to reusable blocks, which can significantly improve programming time.
Templates, on the other hand, are pre-designed structures that provide a framework for specific kinds of projects, such as website layouts, email formats, or documentation. They usually contain placeholders or example content that can be replaced with custom data.
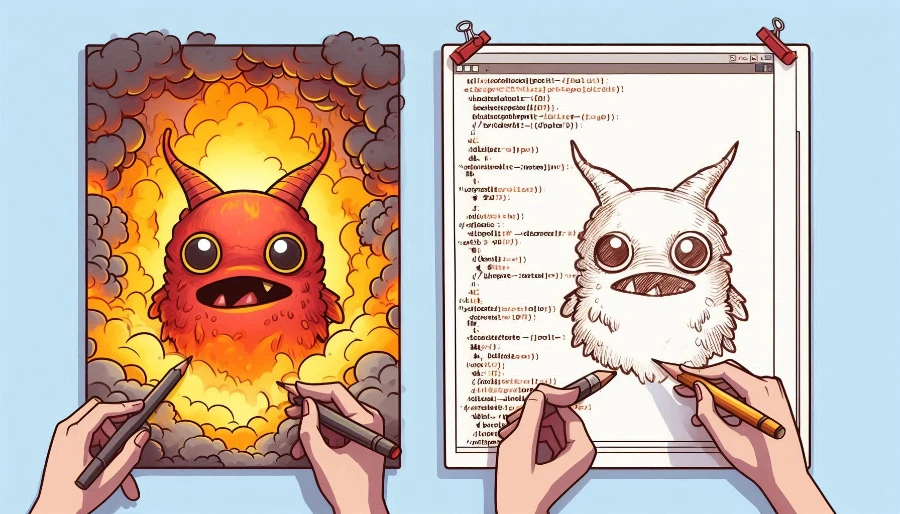
Templates are often more visual and user-focused, providing a starting point for design or content presentation, whereas boilerplate code focuses more on the technical structure and functionality of the application.
Frequently asked questions about Boilerplate code for programmers
What is boilerplate code in programming?
Boilerplate code refers to reusable components and sections that provide programmers with standard structures. Its main purpose is to reduce repetitive setup tasks and save time creating or refining code.
What is the difference between boilerplate code snippets?
Code snippets are small blocks of reusable code that can further optimize an existing project. However, boilerplate code is a bigger infrastructure used for project setups.
Where can I save and edit the boilerplate code?
Every code editor, including the popular VS Code, allows working with, editing, and saving code. The choice is up to your preferences in code editors.